Site
News
Files Visual Basic
Strings
Math
General
Properties
Memory
Methods Search
Testing Inline ASM-VB
Strings
Math
General
Memory Search
Using inline ASM
Submit!
News
Files Visual Basic
Strings
Math
General
Properties
Memory
Methods Search
Testing Inline ASM-VB
Strings
Math
General
Memory Search
Using inline ASM
Submit!
Bitshifting with inline ASMWith bitshifting you can shift bits left or right, effectively multiplying or dividing the number by two.
It's a standard processor operation, and it's quite a common use in C which supports it standardly.
This small piece of code will allow you to do bit shifting (both left and right) easily.
A VB version is also included.
User contributed notes:
It's a standard processor operation, and it's quite a common use in C which supports it standardly.
This small piece of code will allow you to do bit shifting (both left and right) easily.
A VB version is also included.
Public Function asmBitShiftLeft(ByVal Number As Long, ByVal Shift As Long) As Long '#ASM_START ' push ebp ' mov ebp, esp ' ' mov eax, [ebp+8] ; Get first argument ' mov ecx, [ebp+12] ; Get second argument ' shl eax, cl ' mov esp, ebp ;MOV/POP is much faster ' pop ebp ;on 486 and Pentium than Leave ' ret 8 '#ASM_END End Function Public Function asmBitShiftRight(ByVal Number As Long, ByVal Shift As Long) As Long '#ASM_START ' push ebp ' mov ebp, esp ' ' mov eax, [ebp+8] ; Get first argument ' mov ecx, [ebp+12] ; Get second argument ' shr eax, cl ' mov esp, ebp ;MOV/POP is much faster ' pop ebp ;on 486 and Pentium than Leave ' ret 8 '#ASM_END End Function Public Function vbBitShiftLeft(ByVal Number As Long, ByVal Shift As Long) As Long Dim I As Long vbBitShiftLeft = Number For I = 1 To Shift vbBitShiftLeft = vbBitShiftLeft * 2 Next I End Function Public Function vbBitShiftRight(ByVal Number As Long, ByVal Shift As Long) As Long Dim I As Long vbBitShiftRight = Number For I = 1 To Shift vbBitShiftRight = vbBitShiftRight / 2 Next I End Function |
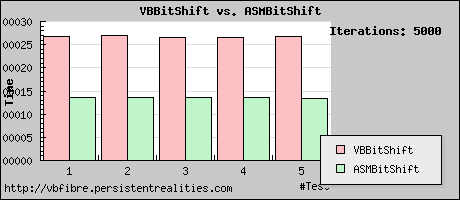
VBBitShift % faster than ASMBitShift | VBBitShift (sec) | ASMBitShift (sec) |
98.1% | 0.000267 | 0.000135 |
100% | 0.00027 | 0.000135 |
96.3% | 0.000265 | 0.000135 |
96.3% | 0.000265 | 0.000135 |
100% | 0.000268 | 0.000134 |
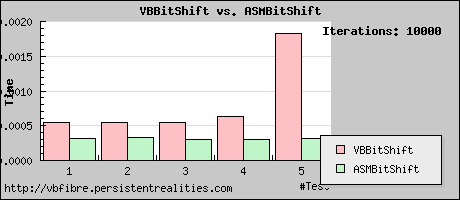
VBBitShift % faster than ASMBitShift | VBBitShift (sec) | ASMBitShift (sec) |
76% | 0.000547 | 0.000311 |
61.9% | 0.000546 | 0.000337 |
78.8% | 0.000546 | 0.000305 |
108% | 0.000634 | 0.000305 |
490.5% | 0.001831 | 0.00031 |
User contributed notes:
Author: Tom (hurendo_kun at hotmail dot com) | Date: 16:06 08/06/2005 |
Just a reminder: bit-shifting only works on integers. If you need to shift a float, you can type-cast it --- visit GameDev and search the forums for "Carmack Inverse Square Root" to find out why. |
|
Author: Randomguy () | Date: 10:12 18/12/2005 |
Uh, is it just me or shouldn't you have just divided once by 2^shift? (calculate that beforehand tho) | |
Author: Spodi () | Date: 05:04 15/04/2007 |
And shouldn't the division be integer-divison, not floating-point division? As your other test on that shows, that makes a significant difference. ;) | |
Author: Almar () | Date: 19:04 15/04/2007 |
Both correct. :). |